0. 개요
포스팅수가 영 부족해서 양 채우려고
옛날에 공부하면서 적었던 자료 정리해서 올린다.
아마 1.3.0 버전에서 동작하는 코드일 것.
variable, autograd 등 없는 버전이다.
- Tensor Creation
- Indexing, Joining, Slicing, Squeezing
- Initialization
- Operations : arithmetic, matrix
1. Tensor Creation
(0) Basic
import torch
int_tensor = torch.IntTensor([[0, 0, 0],[1, 1, 1]])
float_tensor = torch.FloatTensor([[0, 0, 0],[1, 1, 1]])
print(int_tensor)
print(float_tensor)
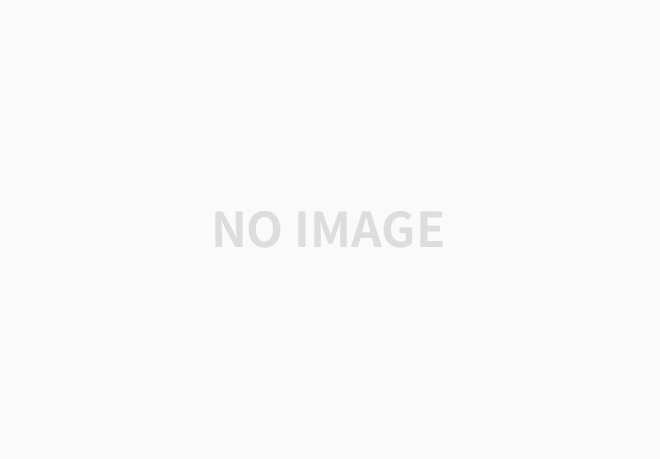
(1) Random Number Generation
import torch
# torch.rand(sizes) -> uniform (0,1)
# torch.randn(sizes) -> Z(0,1)
# torch.randperm(n) -> permutation of 0-n
x = torch.rand(2,3)
y = torch.randn(2,3)
z = torch.randperm(5)
print(x)
print(y)
print(z)
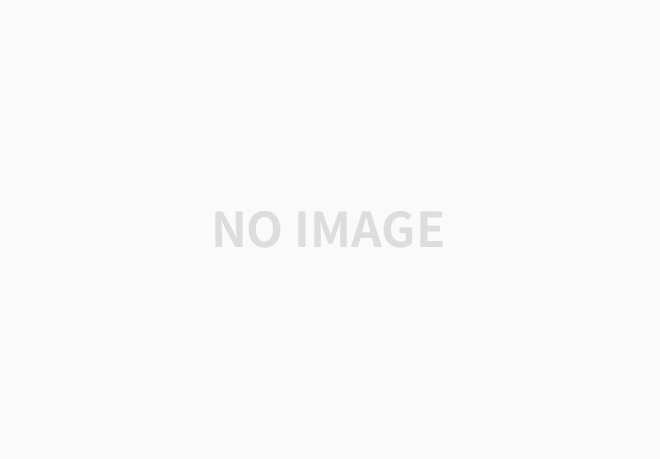
(2) zeros, ones, range
import torch
x = torch.zeros(2,3)
y = torch.ones(2,3)
z = torch.arange(0,3,step=0.5)
print(x)
print(y)
print(z)
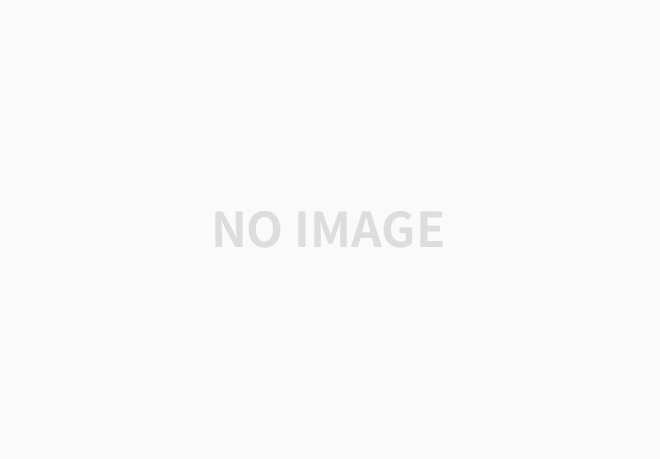
(3) Data Type
#torch.FloatTensor(size or list)
import torch
x = torch.FloatTensor(2,3) # size
y = torch.FloatTensor([2,3]) # list : value itself
z = x.type_as(torch.IntTensor()) # type cast
print(x)
print(y)
print(z)
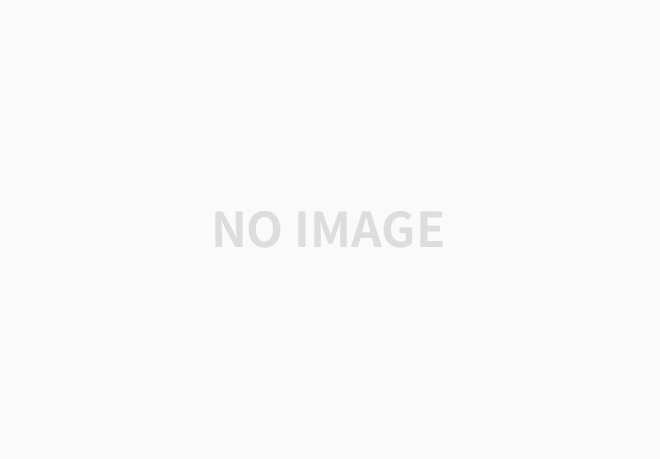
(4) Numpy Casting
import torch
import numpy as np
x = np.ndarray(shape=(2,3), dtype = int, buffer = np.array([1,2,3,4,5,6]))
y = torch.from_numpy(x) # ndarray->tensor
z = y.numpy() # torch->ndarray
print(type(x))
print(type(y))
print(type(z))
print(x==z)
print(x==y)
print(y==z)
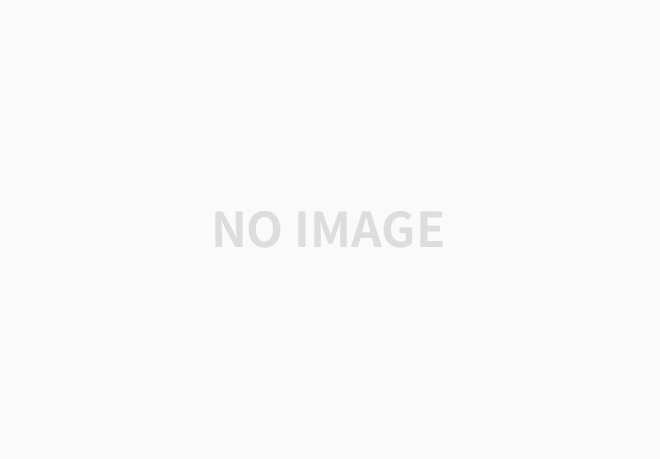
(5) CPU & GPU
import torch
x = torch.FloatTensor([[1,2,3],[4,5,6]])
x_gpu = x.cuda()
x_cpu = x.cpu()
x_gpu2 = x.to('cuda')
x_cpu2 = x.to('cpu')
print(x)
print(x_gpu)
print(x_gpu2)
print(x_cpu)
print(x_cpu2)
print(x==x_cpu)
print(x_cpu==x_cpu2)
print(x_gpu==x_gpu2)
# print(x_cpu==x_gpu) # gives error
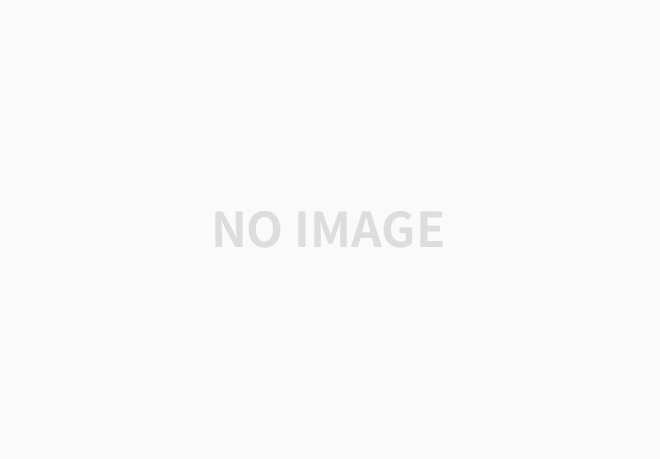
(6) Tensor Size
# tensor.size() -> indexing possible
x = torch.FloatTensor()
y = torch.FloatTensor([[0,0,0],[1,2,3]])
print(x.size())
print(y.size())
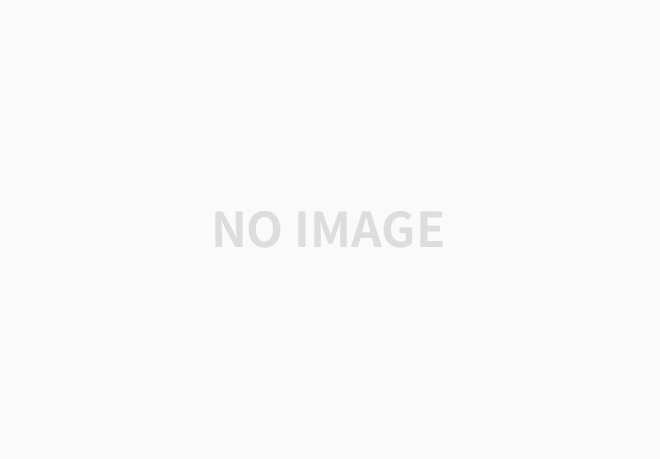
2. Indexing, Joining, Slicing, Squeezing
(1) Indexing
import torch
# torch.index_select(input, dim, index)
x = torch.rand(4,3)
out = torch.index_select(x, 0, torch.LongTensor([0,3]))
print(x)
print(out)
# pythonic indexing
print(x[:,0])
print(x[0:2, 0:2])
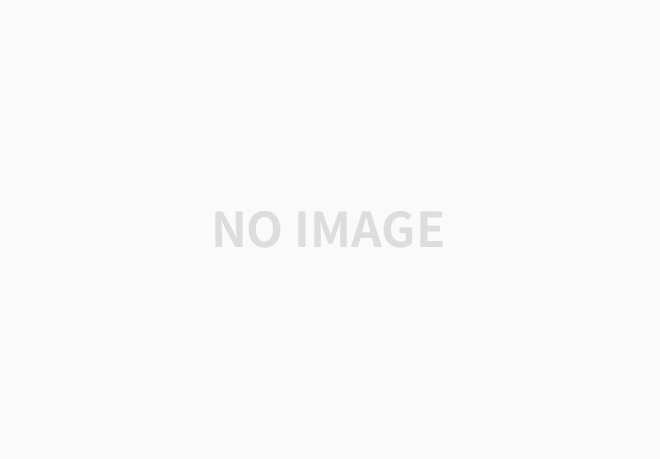
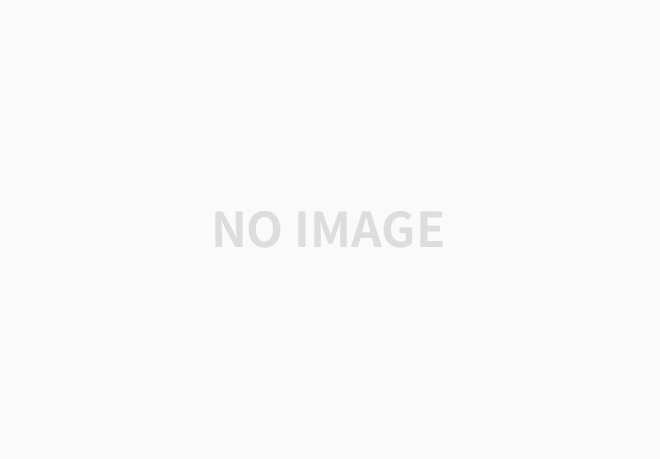
# torch.masked_select(input, mask)
import torch
x = torch.randn(2,3)
mask = torch.BoolTensor([[0,0,1],[0,1,0]])
#mask = torch.ByteTensor([[0,0,1],[0,1,0]]) : uint8
out = torch.masked_select(x, mask)
print(x)
print(mask)
print(out)
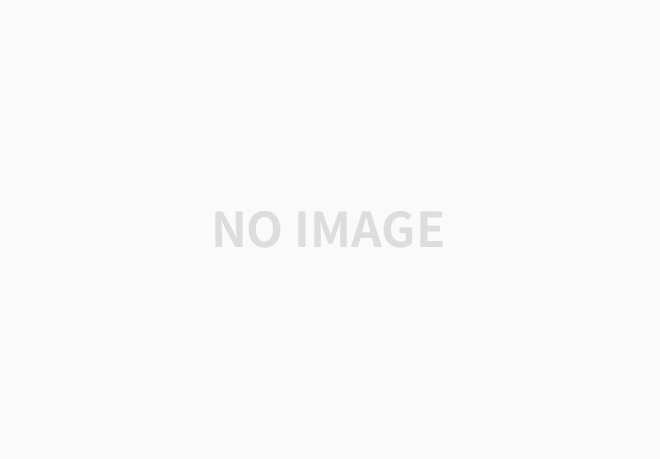
(2) Joining
# torch.cat(seq, dim=0) -> concatenate tensor along dim
import torch
x = torch.FloatTensor([[1,2,3],[4,5,6]])
y = torch.FloatTensor([[-1,-2,-3],[-4,-5,-6]])
z1 = torch.cat([x,y], dim=0)
z2 = torch.cat([x,y], dim=1)
print(z1)
print(z2)
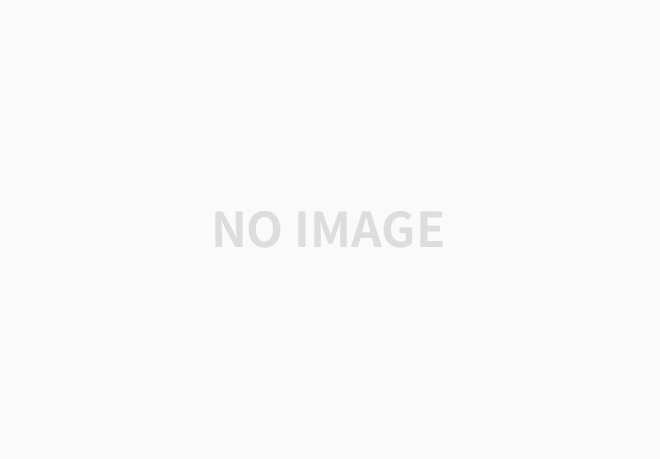
# torch.stack(seq, dim=0) -> new dimension
import torch
x = torch.FloatTensor([[1,2,3],[4,5,6]])
x_stack1 = torch.stack([x,x,x,x], dim=0)
x_stack2 = torch.stack([x,x,x,x], dim=1)
x_stack3 = torch.stack([x,x,x,x], dim=2)
print(x_stack1)
print(x_stack2)
print(x_stack3)
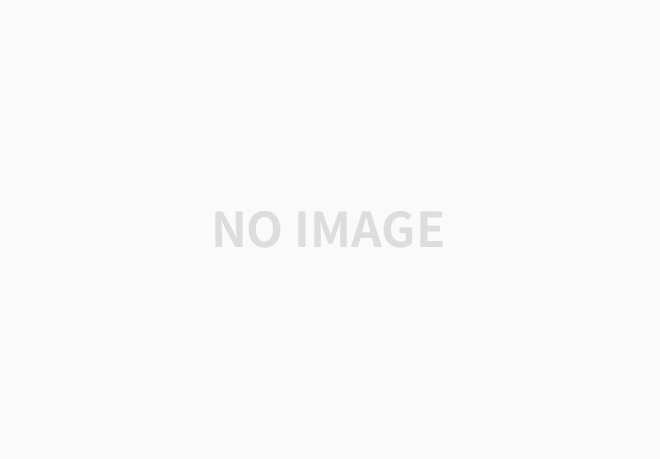
(3) Slicing
# torch.chunk(tensor, chunks, dim=0)
import torch
x = torch.FloatTensor([[1,2,3],[4,5,6]])
y = torch.FloatTensor([[-1,-2,-3],[-4,-5,-6]])
z = torch.cat([x,y], dim=0)
x1, x2 = torch.chunk(z, 2, dim=0)
y1, y2, y3 = torch.chunk(z, 3, dim=1)
print(z1)
print(x1)
print(x2)
print(y1)
print(y2)
print(y3)
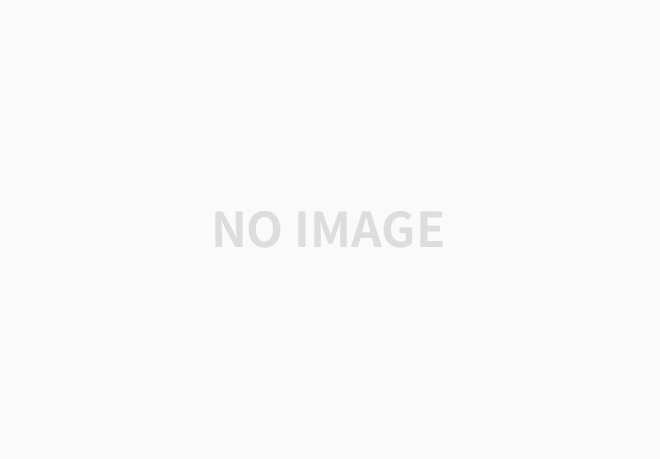
# torch.split(tensor, split_size, dim=0) -> with specific size
import torch
x = torch.FloatTensor([[1,2,3],[4,5,6]])
y = torch.FloatTensor([[-1,-2,-3],[-4,-5,-6]])
z1 = torch.cat([x,y], dim=0)
x1, x2 = torch.split(z1, 2, dim=0)
y1, y2 = torch.split(z1, 2, dim=1)
print(z1)
print(x1)
print(x2)
print(y1)
print(y2)
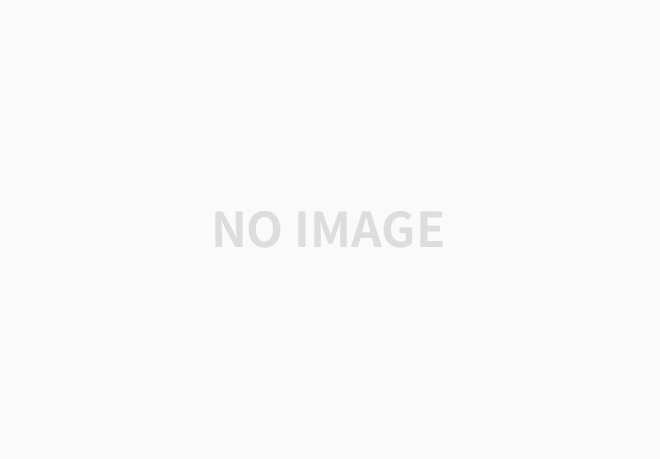
(4) Squeezing & Unsqueezing
# torch.squeeze(input, dim=None) -> reduce dim by 1
import torch
x = torch.FloatTensor(10,1,3,1,4)
y = torch.squeeze(x)
print(x.size())
print(y.size())
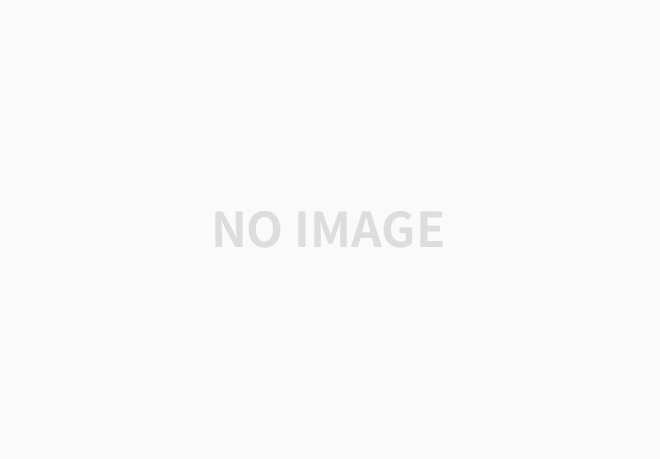
# torch.unsqueeze(input, dim=None) -> add dim by 1
import torch
x = torch.FloatTensor(10, 3, 4)
y = torch.unsqueeze(x, dim=0)
z = torch.stack([x], dim=0)
print(x.size())
print(y.size())
print(z.size())
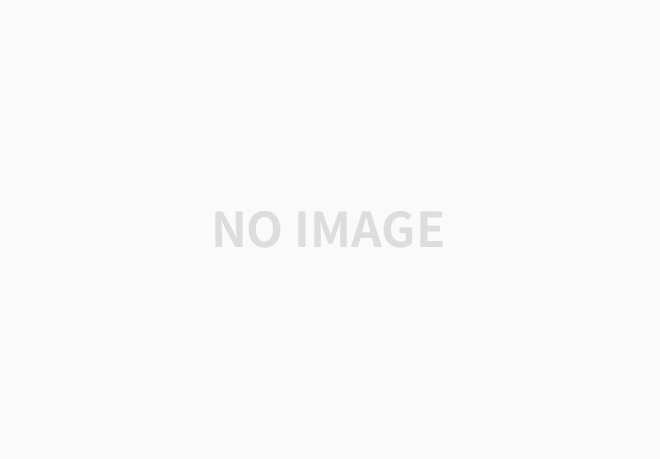
3. Initialization
import torch
import torch.nn.init as init
x1 = init.uniform_(torch.FloatTensor(3,4), a=0, b=9)
x2 = init.normal_(torch.FloatTensor(3,4), std=0.2)
x3 = init.constant_(torch.FloatTensor(3,4), 3.141592)
print(x1)
print(x2)
print(x3)
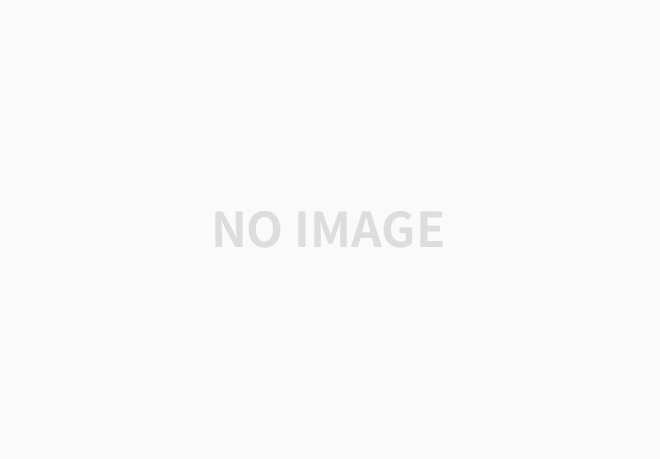
* init.xxx_ 메서드들은 반환값과 관계 없이 input tensor를 initialize한다. 즉, 위와 같은 작위적인 상황이 아니면 x1= 부분은 필요없다.
4. Operations
(1) Arithmetic
# torch.add()
import torch
x = torch.FloatTensor([[1,2,3],[4,5,6]])
y = torch.FloatTensor([[-1,-2,-3],[-4,-5,-6]])
z1 = torch.add(x,y)
z2 = x+y
print(z1)
print(z2)
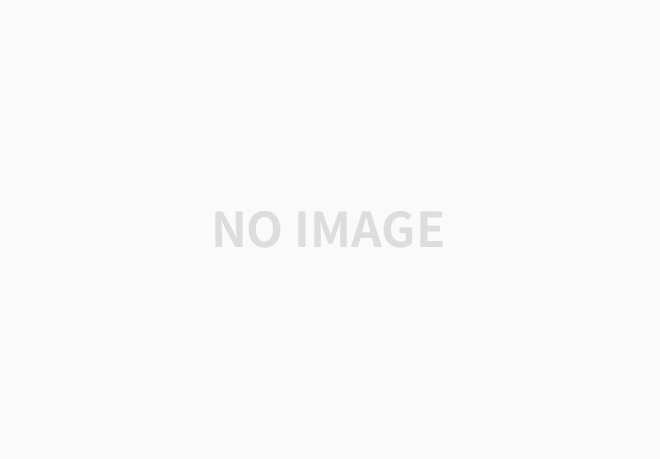
# torch.sub() with broadcasting
x = torch.FloatTensor([[1,2,3],[4,5,6]]) # size (2,3)
y = 10 # size (,) -> casted to size(2,3) when added
z1 = torch.sub(x,y)
z2 = x-y
print(z1)
print(z2)
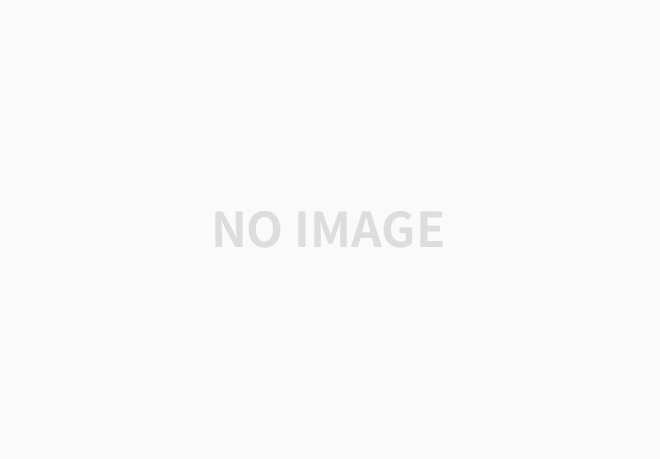
# torch.mul() -> elementwise
import torch
x = torch.FloatTensor([[1,2,3],[4,5,6]])
y1 = torch.FloatTensor([[1,2,3],[4,5,6]])
y2 = 10
z1 = torch.mul(x,y1)
z2 = torch.mul(x,y2)
print(z1)
print(z2)
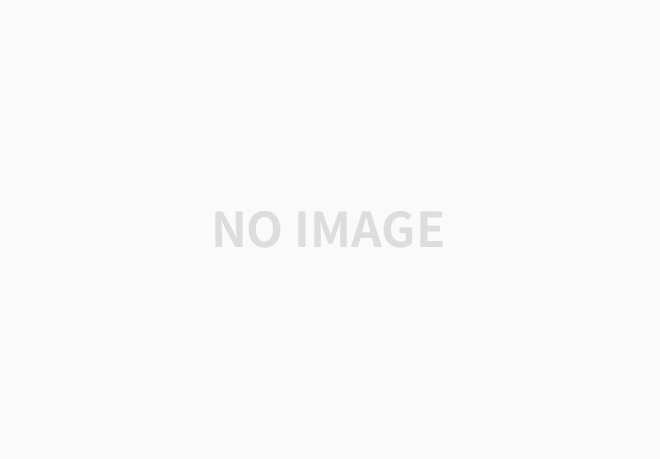
# torch.div() -> size better match
import torch
x = torch.FloatTensor([[1,2,3],[4,5,6]])
y = 5
z = torch.div(x,y)
print(z)
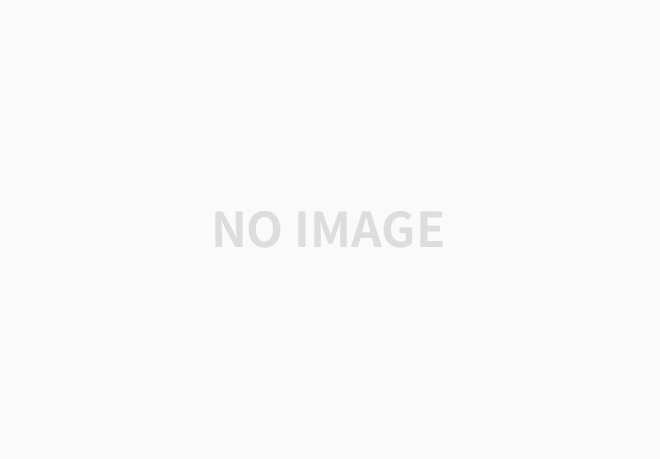
(2) Other Math Operations
# torch.pow(input, exp)
import torch
x = torch.FloatTensor(3,4)
y = torch.pow(x,2)
z = x**2
print(y)
print(z)
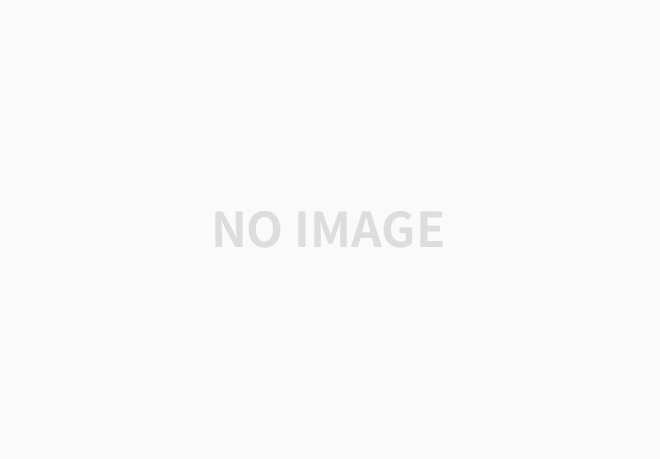
# torch.exp(tensor, out=None)
import torch
x = torch.FloatTensor(3,4)
y = torch.exp(x)
print(y)
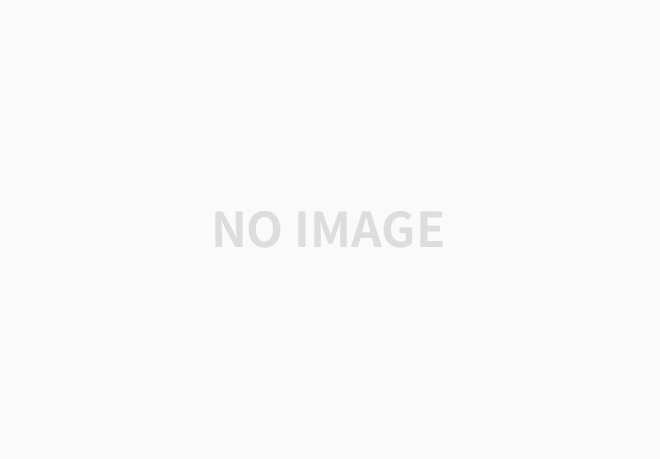
# torch.log(tensor, out=None)
import torch
x = torch.FloatTensor(3,4)
y = torch.log(x)
print(y)
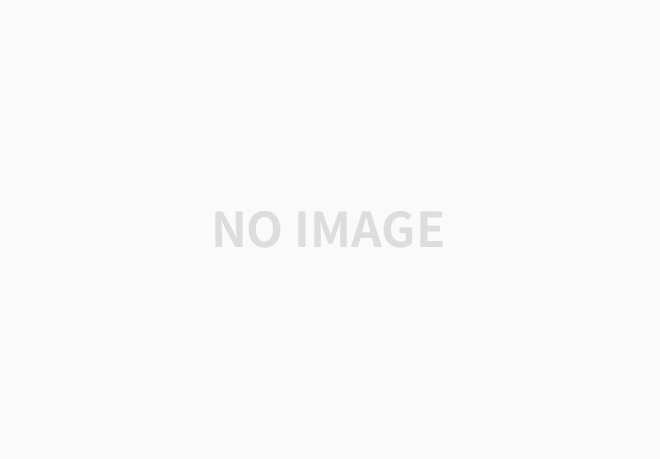
(3) Matrix Operations
# torch.mm(mat1, mat2) -> matrix only (no tensor)
import torch
x = torch.FloatTensor(3,4)
y = torch.FloatTensor(4,1)
z = torch.mm(x,y)
print(z.size())
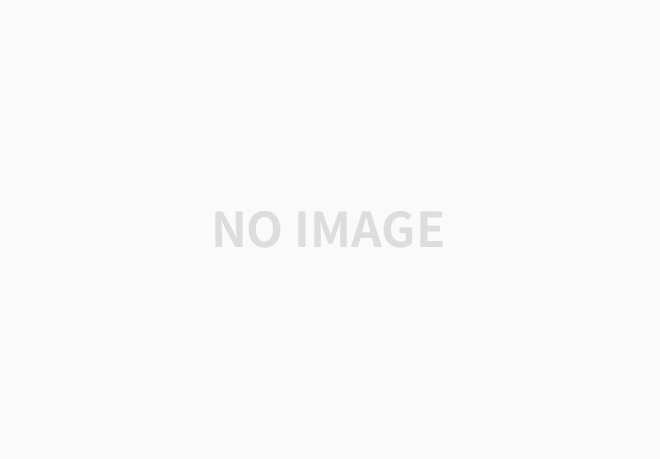
# torch.bmm(batch1, batch2)
import torch
x = torch.FloatTensor(10,3,4)
y = torch.FloatTensor(10,4,1)
z = torch.bmm(x,y)
print(z.size())
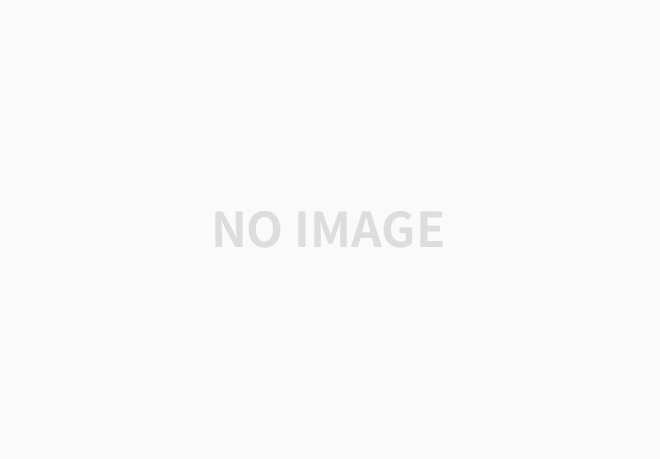
# torch.dot(tensor1, tensor2)
import torch
x = torch.FloatTensor(3)
y = torch.FloatTensor(3)
z = torch.dot(x,y)
print(x)
print(y)
print(z)
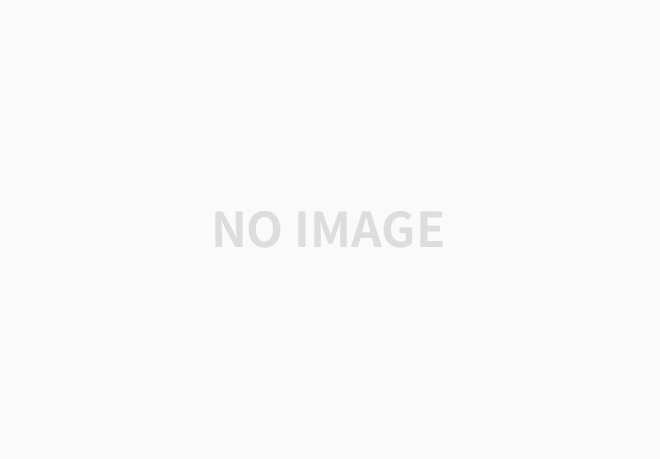
** dot 연산은 1D tensor의 내적이고, mul 연산은 원소별 곱이다. 즉, torch.sum(torch.mul(1D,1D)) 의 결과는 torch.dot과 같다.
# torch.t(matrix)
import torch
x = torch.FloatTensor(3,4)
y = torch.t(x)
z = x.t()
print(y.size())
print(z.size())
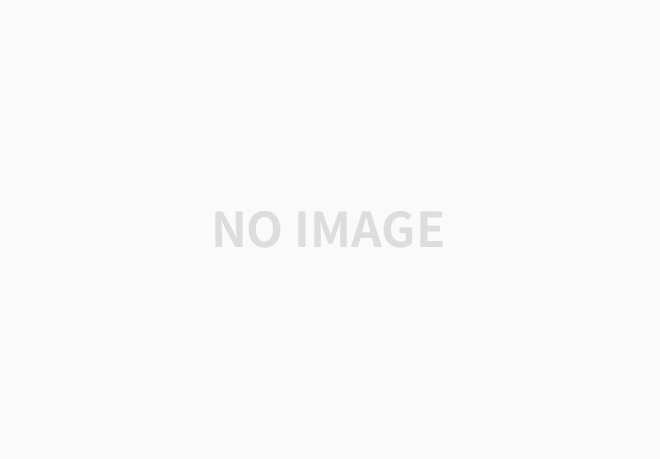
numpy는 x.T 인 것에 비해 좀 불편하다.
# torch.transpose(tensor, dim0, dim1)
import torch
x = torch.FloatTensor(10,3,4)
y = torch.transpose(x, 1, 2)
z = x.transpose(1,2)
print(y.size())
print(z.size())
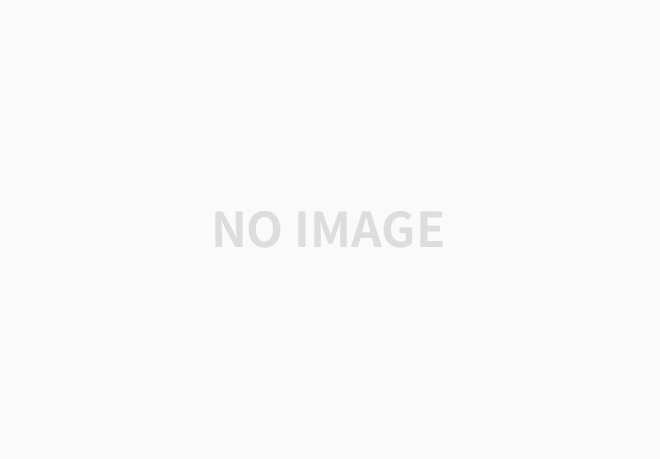
# torch.eig(a, eigenvectors=False) -> eigenvalue, eigenvector
import torch
x = torch.FloatTensor(4,4)
eig_val, eig_vec = torch.eig(x, True)
print(eig_val)
print(eig_vec)
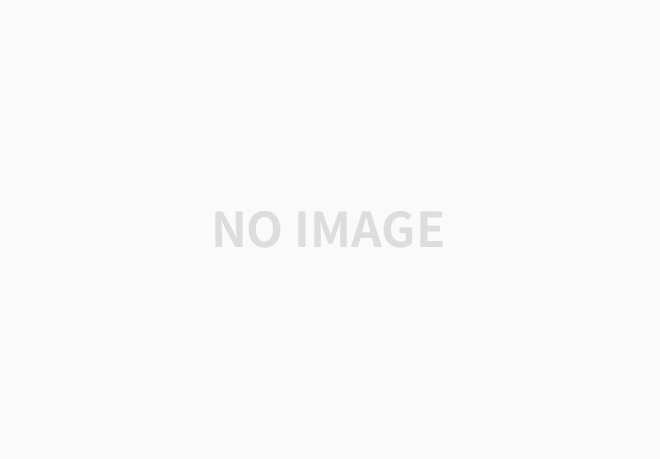
'SW > DeepLearning' 카테고리의 다른 글
[Deep Learning] L1 Regularization의 Gradient에 관한 소고 (0) | 2020.07.02 |
---|---|
[PyTorch] PyTorch Basic - 기본 틀 (0) | 2020.06.29 |
[Pytorch] Pytorch로 ResNet bottleneck 만들기 (0) | 2020.06.27 |